递归DP-金币组合最少金币数
package com.jiucaiyuan.net.question; /** * @Author jiucaiyuan 2022/6/5 11:33 * @mail services@jiucaiyuan.net */ public class CoinAmount { /** * 暴力递归 * 给一个正数数组,每个数表示一枚硬币的面值,给定一个数值,从硬币中选择得到这个金额的最少硬币数 * 比如指定硬币有[1,4,7,2,4,3,5,1,3] * 指定金额10,那么最少硬币数是7+3,两枚硬币,返回2 * * @param arr 硬币,arr.length硬币个数,arr[index]表示index硬币金额 * @param amount 组合最终的金额 * @return 组合最终金额的最少硬币数 */ public static int minCoinsCountV1(int[] arr, int amount) { return processV1(arr, 0, amount); } public static int processV1(int[] arr, int index, int rest) { //超额了 if (rest < 0) { return -1; } //金额正好 if (rest == 0) { return 0; } //钱还有,但是硬币没了 if (index == arr.length) { return -1; } //钱还有,硬币也还有 //当前硬币不选择,往后尝试 int p1 = processV1(arr, index + 1, rest); //当前硬币选择,往后尝试 int p2Next = processV1(arr, index + 1, rest - arr[index]); //如果后续尝试后,发现金额超了,不能组成目标金额 if (p1 == -1 && p2Next == -1) { return -1; } //如果 if (p1 == -1) { return p2Next + 1; } if (p2Next == -1) { return p1; } return Math.min(p1, p2Next + 1); } /** * 暴力递归添加缓存,去掉重复计算,空间换时间 * <p> * 给一个正数数组,每个数表示一枚硬币的面值,给定一个数值,从硬币中选择得到这个金额的最少硬币数 * 比如指定硬币有[1,4,7,2,4,3,5,1,3] * 指定金额10,那么最少硬币数是7+3,两枚硬币,返回2 * * @param arr 硬币,arr.length硬币个数,arr[index]表示index硬币金额 * @param amount 组合最终的金额 * @return 组合最终金额的最少硬币数 */ public static int minCoinsCountV2(int[] arr, int amount) { int[][] dp = new int[arr.length + 1][amount + 1]; for (int i = 0; i <= arr.length; i++) { for (int j = 0; j <= amount; j++) { dp[i][j] = -2;//-2表示没有计算过 -1表示计算过,无效 } } return processV2(arr, 0, amount, dp); } ; public static int processV2(int[] arr, int index, int rest, int[][] dp) { //超额了 if (rest < 0) { return -1; } if (dp[index][rest] != -2) { return dp[index][rest]; } if (rest == 0) { //金额正好 dp[index][rest] = 0; } else if (index == arr.length) { //钱还有(amount > 0) // 没硬币了 dp[index][rest] = -1; } else { //硬币也还有 //当前硬币不选择,往后尝试 int p1 = processV2(arr, index + 1, rest, dp); //当前硬币选择,往后尝试 int p2Next = processV2(arr, index + 1, rest - arr[index], dp); //如果后续尝试后,发现金额超了,不能组成目标金额 if (p1 == -1 && p2Next == -1) { dp[index][rest] = -1; } else if (p1 == -1) { dp[index][rest] = p2Next + 1; } else if (p2Next == -1) { dp[index][rest] = p1; } else { dp[index][rest] = Math.min(p1, p2Next + 1); } } return dp[index][rest]; } /** * 动态规划版本 * <p> * 给一个正数数组,每个数表示一枚硬币的面值,给定一个数值,从硬币中选择得到这个金额的最少硬币数 * 比如指定硬币有[1,4,7,2,4,3,5,1,3] * 指定金额10,那么最少硬币数是7+3,两枚硬币,返回2 * * @param arr 硬币,arr.length硬币个数,arr[index]表示index硬币金额 * @param amount 组合最终的金额 * @return 组合最终金额的最少硬币数 */ public static int minCoinsCountV3(int[] arr, int amount) { int[][] dp = new int[arr.length + 1][amount + 1]; for (int i = 0; i <= arr.length; i++) { dp[i][0] = 0; } for (int col = 1; col <= amount; col++) { dp[arr.length][col] = -1; } for (int index = arr.length - 1; index >= 0; index--) { for (int rest = 1; rest <= amount; rest++) { //过程改自递归调用的process方法 int p1 = dp[index + 1][rest]; int p2Next = rest - arr[index] >= 0 ? dp[index + 1][rest - arr[index]] : -1; if (p1 == -1 && p2Next == -1) { dp[index][rest] = -1; } else if (p1 == -1) { dp[index][rest] = p2Next + 1; } else if (p2Next == -1) { dp[index][rest] = p1; } else { dp[index][rest] = Math.min(p1, p2Next + 1); } } } return dp[0][amount]; } //for test public static int[] generateRandomArray(int length, int max) { int[] arr = new int[(int) (Math.random() * length) + 1]; for (int i = 0; i < arr.length; i++) { arr[i] = (int) (Math.random() * max) + 1; } return arr; } public static void main(String[] args) { int[] array = {4, 9, 10, 4}; minCoinsCountV2(array, 17); int len = 10; int max = 10; int testTimes = 100000; for (int i = 0; i < testTimes; i++) { int[] arr = generateRandomArray(len, max); int amount = (int) (Math.random() * 3 * max) + max; System.out.println("------------------------------" + i); System.out.println(print(arr) + "<----->" + amount); int minCoinsCountV1 = minCoinsCountV1(arr, amount); int minCoinsCountV2 = minCoinsCountV2(arr, amount); int minCoinsCountV3 = minCoinsCountV3(arr, amount); System.out.println(minCoinsCountV1 + "<----->" + minCoinsCountV2 + "<----->" + minCoinsCountV3); if (minCoinsCountV1 != minCoinsCountV2 || minCoinsCountV1 != minCoinsCountV3) { System.out.println("goooooooood!"); break; } } } private static String print(int[] arr) { StringBuffer sb = new StringBuffer(); for (int a : arr) { if (sb.length() == 0) { sb.append("[").append(a); } else { sb.append(",").append(a); } } sb.append("]"); return sb.toString(); } }
日历
个人资料
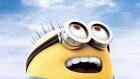
diaba 寻求合作请留言或联系mail: services@jiucaiyuan.net
链接
最新文章
存档
- 2025年4月(17)
- 2025年3月(25)
- 2025年2月(20)
- 2025年1月(2)
- 2024年10月(1)
- 2024年8月(2)
- 2024年6月(4)
- 2024年5月(1)
- 2023年7月(1)
- 2022年10月(1)
- 2022年8月(1)
- 2022年6月(11)
- 2022年5月(6)
- 2022年4月(33)
- 2022年3月(26)
- 2021年3月(1)
- 2020年9月(2)
- 2018年8月(1)
- 2018年3月(1)
- 2017年3月(3)
- 2017年2月(6)
- 2016年12月(3)
- 2016年11月(2)
- 2016年10月(1)
- 2016年9月(3)
- 2016年8月(4)
- 2016年7月(3)
- 2016年6月(4)
- 2016年5月(7)
- 2016年4月(9)
- 2016年3月(4)
- 2016年2月(5)
- 2016年1月(17)
- 2015年12月(15)
- 2015年11月(11)
- 2015年10月(6)
- 2015年9月(11)
- 2015年8月(8)
分类
热门文章
- SpringMVC:Null ModelAndView returned to DispatcherServlet with name 'applicationContext': assuming HandlerAdapter completed request handling
- Mac-删除卸载GlobalProtect
- java.lang.SecurityException: JCE cannot authenticate the provider BC
- MyBatis-Improper inline parameter map format. Should be: #{propName,attr1=val1,attr2=val2}
- Idea之支持lombok编译
标签
最新评论
- logisqykyk
Javassist分析、编辑和创建jav... - xxedgtb
Redis—常见参数配置 - 韭菜园 ... - wdgpjxydo
SpringMVC:Null Model... - rllzzwocp
Mysql存储引擎MyISAM和Inno... - dpkgmbfjh
SpringMVC:Null Model... - tzklbzpj
SpringMVC:Null Model... - bqwrhszmo
MyBatis-Improper inl... - 乐谱吧
good非常好 - diaba
@diaba:应该说是“时间的度量依据”... - diaba
如果速度增加接近光速、等于光速、甚至大于...
最新微语
- 在每件事情上花费的东西,就是生命的一部分,而我们花费的这些东西要求立即得到回报,或者在一个长时间以后得到回报。
2025-01-23 15:46
- 诺曼·文森特说:“并不是你认为自己是什么样的人,你就是什么样的人。但是你的思想是什么样,你就是什么样的人。”
2025-01-23 15:44
- 从今天起,做一个幸福的人。喂马,砍柴,(思想)周游世界
2022-03-21 23:31
- 2022.03.02 23:37:59
2022-03-02 23:38
- 几近崩溃后,找到解决方法,总是那么豁然开朗!所以遇到问题要坚持!
2018-07-18 10:49
发表评论: