算法-二叉树-判断搜索二叉树
package com.jiucaiyuan.net.algrithm.tree; import java.util.Stack; /** * <pre> * 【问题】判断一棵树是否是搜索二叉树(Binary Search Tree),(又:二叉搜索树,二叉排序树) * 【概念】搜索二叉树:树的左子树比头结点小,右子树比头结点大(左子<头<右子) * 【思路】通过二叉树的中序遍历,判断树中节点一直是升序的,不出现降序即可 * </pre> * * @Author jiucaiyuan 2022/4/10 16:42 * @mail services@jiucaiyuan.net */ public class IsBST { /** * 判断是搜索二叉树(递归套路实现) * * @param root * @return */ public static boolean isBst(Node<Integer> root) { return process(root).isBst; } /** * <pre> * 使用二叉树递归套路来处理 * 1.基本case处理 * 2.询问左子树结果 * 3.询问右子树结果 * 4.处理当前节点是否满足,返回最终结果 * </pre> * * @param x * @return */ public static ResultData process(Node<Integer> x) { if (x == null) { //如果返回ResultData,最大值和最小值不好设置,所以返回null,分情况处理 return null; } ResultData leftResult = process(x.left); ResultData rightResult = process(x.right); //组织返回结果使用 int minValue = x.value; int maxValue = x.value; if (leftResult != null) { minValue = Math.min(minValue, leftResult.minValue); maxValue = Math.max(maxValue, leftResult.maxValue); } if (rightResult != null) { minValue = Math.min(minValue, rightResult.minValue); maxValue = Math.max(maxValue, rightResult.maxValue); } //当前是否符合搜索二叉树条件 boolean isBst = true; if (leftResult != null && (!leftResult.isBst || leftResult.maxValue >= x.value)) { isBst = false; } if (rightResult != null && (!rightResult.isBst || x.value >= rightResult.minValue)) { isBst = false; } return new ResultData(isBst, minValue, maxValue); } static class ResultData { boolean isBst; int minValue; int maxValue; ResultData(boolean isBst, int min, int max) { isBst = isBst; minValue = min; maxValue = max; } } //中序遍历实现,每次递归比较之前处理的值 private static int preValue = Integer.MIN_VALUE; /** * 中序遍历-递归 */ public static boolean isBstRecur(Node<Integer> root) { if (root == null) { return true; } //左是否是搜索二叉树 boolean isLeftBst = isBstRecur(root.left); if (!isLeftBst) { return false; } //如果当前节点不大于前一个处理的值,非搜索二叉树 if (root.value <= preValue) { return false; } else { //记录前一个处理的值 preValue = root.value; } //判断右是否是搜索二叉树 return isBstRecur(root.right); } /** * 中序遍历-非递归 * 处理过程:指定root节点的整棵树左边树进栈,判断是否满足搜索二叉树,针对弹出节点右树重复该节点的左树进栈 */ public static boolean isBstUnRecur(Node<Integer> root) { if (root != null) { int preValue = Integer.MIN_VALUE; Stack<Node> stack = new Stack<>(); while (!stack.isEmpty() || root != null) { //不停的把左边树进栈 if (root != null) { stack.push(root); root = root.left; } else { //如果没有左树了,弹出,处理该节点的右子树(再次进入while时还是处理该节点的左边树进栈) root = stack.pop(); if (root.value <= preValue) { return false; } else { preValue = root.value; } root = root.right; } } } return true; } }
日历
个人资料
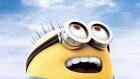
diaba 寻求合作请留言或联系mail: services@jiucaiyuan.net
链接
最新文章
存档
- 2025年4月(17)
- 2025年3月(25)
- 2025年2月(20)
- 2025年1月(2)
- 2024年10月(1)
- 2024年8月(2)
- 2024年6月(4)
- 2024年5月(1)
- 2023年7月(1)
- 2022年10月(1)
- 2022年8月(1)
- 2022年6月(11)
- 2022年5月(6)
- 2022年4月(33)
- 2022年3月(26)
- 2021年3月(1)
- 2020年9月(2)
- 2018年8月(1)
- 2018年3月(1)
- 2017年3月(3)
- 2017年2月(6)
- 2016年12月(3)
- 2016年11月(2)
- 2016年10月(1)
- 2016年9月(3)
- 2016年8月(4)
- 2016年7月(3)
- 2016年6月(4)
- 2016年5月(7)
- 2016年4月(9)
- 2016年3月(4)
- 2016年2月(5)
- 2016年1月(17)
- 2015年12月(15)
- 2015年11月(11)
- 2015年10月(6)
- 2015年9月(11)
- 2015年8月(8)
分类
热门文章
- SpringMVC:Null ModelAndView returned to DispatcherServlet with name 'applicationContext': assuming HandlerAdapter completed request handling
- Mac-删除卸载GlobalProtect
- java.lang.SecurityException: JCE cannot authenticate the provider BC
- MyBatis-Improper inline parameter map format. Should be: #{propName,attr1=val1,attr2=val2}
- Idea之支持lombok编译
标签
最新评论
- logisqykyk
Javassist分析、编辑和创建jav... - xxedgtb
Redis—常见参数配置 - 韭菜园 ... - wdgpjxydo
SpringMVC:Null Model... - rllzzwocp
Mysql存储引擎MyISAM和Inno... - dpkgmbfjh
SpringMVC:Null Model... - tzklbzpj
SpringMVC:Null Model... - bqwrhszmo
MyBatis-Improper inl... - 乐谱吧
good非常好 - diaba
@diaba:应该说是“时间的度量依据”... - diaba
如果速度增加接近光速、等于光速、甚至大于...
最新微语
- 在每件事情上花费的东西,就是生命的一部分,而我们花费的这些东西要求立即得到回报,或者在一个长时间以后得到回报。
2025-01-23 15:46
- 诺曼·文森特说:“并不是你认为自己是什么样的人,你就是什么样的人。但是你的思想是什么样,你就是什么样的人。”
2025-01-23 15:44
- 从今天起,做一个幸福的人。喂马,砍柴,(思想)周游世界
2022-03-21 23:31
- 2022.03.02 23:37:59
2022-03-02 23:38
- 几近崩溃后,找到解决方法,总是那么豁然开朗!所以遇到问题要坚持!
2018-07-18 10:49
发表评论: